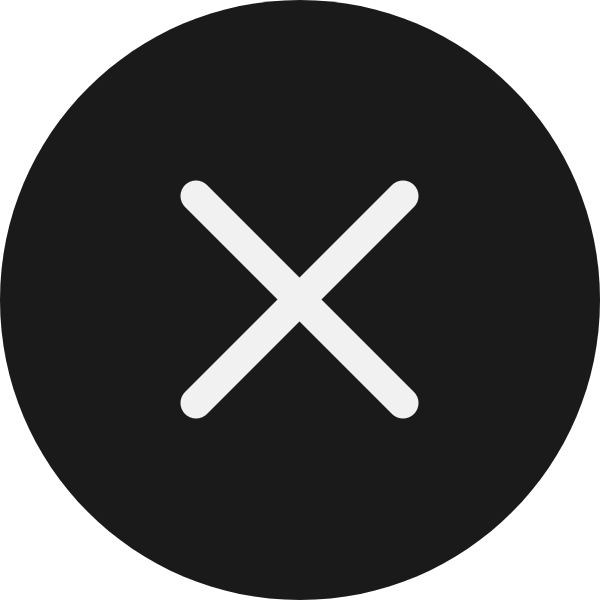
gittech. site
for different kinds of informations and explorations.
Graphina: A High-Level Graph Data Science Library for Rust

Graphina
Graphina is a graph data science library for Rust. It provides the common data structures and algorithms used for analyzing the graphs of real-world networks such as social, transportation, and biological networks.
Compared to other Rust graph libraries like petgraph and rustworkx, Graphina aims to provide a more high-level API and a wide range of ready-to-use algorithms for network analysis and graph mining tasks. The main goal is to make Graphina as feature-rich as NetworkX, but with the performance of Rust.
Additionally, PyGraphina Python library allows users to use Graphina in Python. Check out pygraphina directory for more details.
[!IMPORTANT] Graphina is in the early stages of development, so breaking changes may occur. Bugs and API inconsistencies are also expected, and the algorithms may not yet be optimized for performance.
Structure
Graphina consists of two main parts: a core library and extensions. The core library provides the basic data structures and algorithms for working with graphs. The extensions are modules outside the core library that contain more advanced algorithms for specific tasks like community detection, link prediction, and calculating node and edge centrality scores.
The extensions are independent of each other. However, they depend on the core library for the basic graph operations.
Graphina Core
Module | Feature/Algorithm | Status | Notes |
---|---|---|---|
Types |
|
Tested | Graph types that Graphina supports |
Exceptions |
|
Tested | Custom error types for Graphina |
IO |
|
Tested | I/O routines for reading/writing graph data |
Generators |
|
Tested | Graph generators for random and structured graphs |
Paths |
|
Tested | Shortest paths algorithms |
MST |
|
Tested | Minimum spanning tree algorithms |
Traversal |
|
Tested | Graph traversal algorithms |
Extensions
Module | Feature/Algorithm | Status | Notes |
---|---|---|---|
Centrality |
|
Centrality measures | |
Links |
|
Link prediction algorithms | |
Community |
|
Community detection and clustering algorithms | |
Approximation |
|
Approximations and heuristic methods for NP‑hard problems |
[!NOTE] Status shows whether the module is
Tested
andBenchmarked
. Empty status means the module is implemented but not tested and benchmarked yet.
Installation
cargo add graphina
Graphina requires Rust 1.83 or later.
Documentation
See the docs for the latest documentation.
Check out the docs.rs/graphina for the latest API documentation.
Simple Example
use graphina::core::types::Graph;
fn main() {
// Create a new undirected graph
let mut graph = Graph::new();
// Add nodes and edges to the graph
let n0 = graph.add_node(1);
let n1 = graph.add_node(1);
let n2 = graph.add_node(2);
let n3 = graph.add_node(3);
graph.add_edge(n0, n1, 1.0);
graph.add_edge(n1, n2, 1.0);
graph.add_edge(n2, n3, 1.0);
// Get the neighbors of node 1
for neighbor in graph.neighbors(n1) {
println!("Node 1 has neighbor: {}", neighbor.index());
}
}
See the tests directory for more usage examples.
Contributing
See CONTRIBUTING.md for details on how to make a contribution.
Logo
The mascot is named "Graphina the Dinosaur". As the name implies, she's a dinosaur, however, she herself thinks she's a dragon. The logo was created using Gimp, ComfyUI, and a Flux Schnell v2 model.
Licensing
Graphina is licensed under either of these:
- MIT License (LICENSE-MIT)
- Apache License, Version 2.0 (LICENSE-APACHE)
PyGraphina is licensed under the MIT License (LICENSE).